Welcome
Bringing the best SwiftUI features to your React Native app 🚀
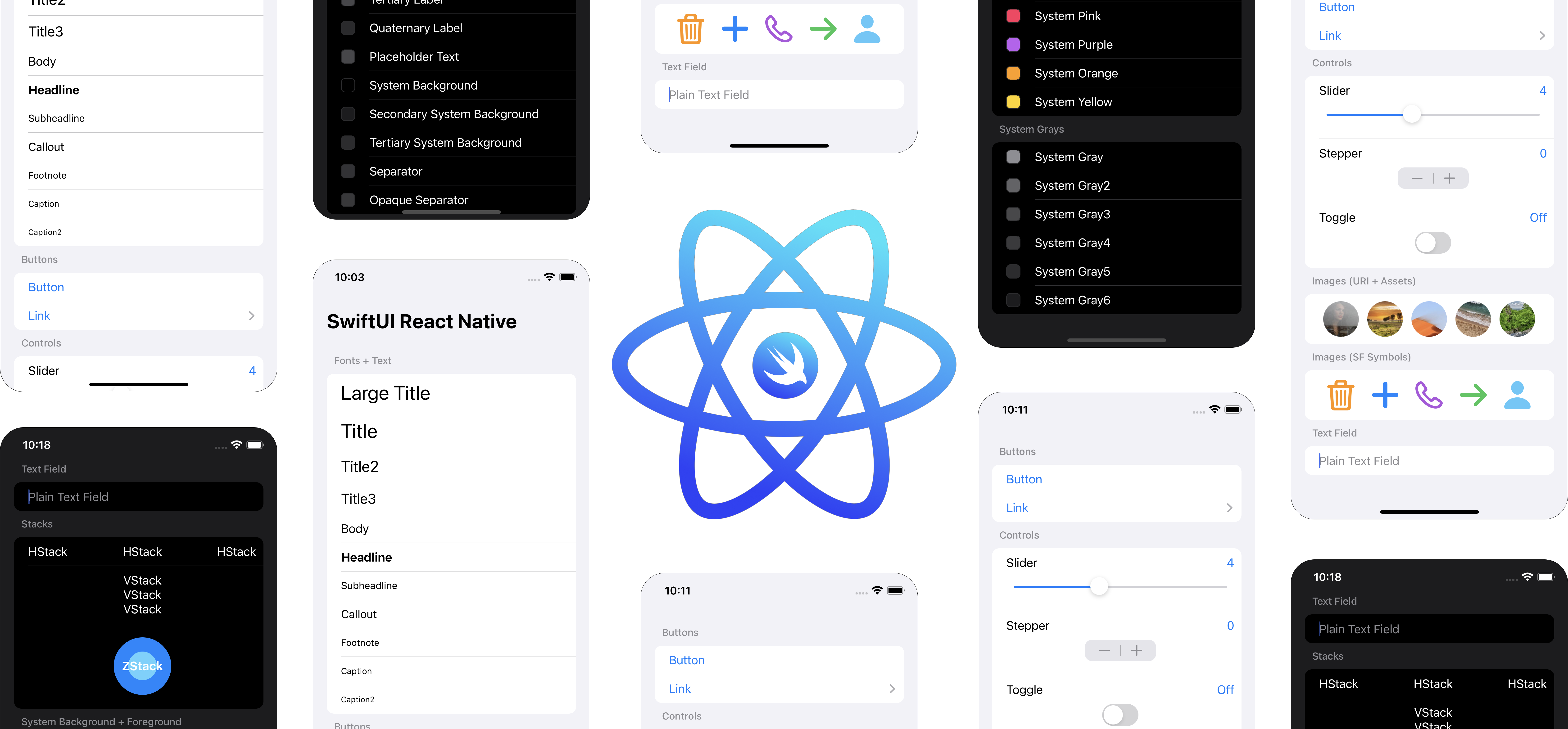
At a Glance​
This library provides you with a set of SwiftUI primitives that you can use in your React Native app.
- Real SwiftUI native views
- SwiftUI Modifiers as props
- Experimental API
note
This is not a cross-platform library. These components will only work on iOS.
Usage​
- swiftui-react-native
- SwiftUI
- React Native
import {
VStack,
Text,
TextField,
Button,
useBinding,
} from 'swiftui-react-native';
function App() {
const text = useBinding('');
return (
<VStack aligment="leading" background="blue" padding={{ leading: 30 }}>
<Text font="title">Some cool text</Text>
<TextField text={text} placeholder="Name" />
<Button title="Click the cool button" action={doSomething} />
</VStack>
);
}
import SwiftUI
struct App: View {
@State var text = ""
var body: some View {
VStack(alignment: .leading) {
Text("Some cool text")
.font(.title)
TextField("Name", text: $text)
Button(action: doSomething) {
Text("Click the cool button").bold()
}
}
.background(.blue)
.padding(.leading, 30)
}
}
import React, { useState } from 'react';
import {
View,
Text,
TextInput,
TouchableOpacity,
StyleSheet,
} from 'react-native';
function App() {
const [text, setText] = useState('');
return (
<View style={styles.container}>
<Text style={styles.title}>Some cool text</Text>
<TextInput
placeholder="Name"
value={text}
onChangeText={(text) => setText(text)}
/>
<TouchableOpacity onPress={doSomething}>
<Text style={styles.buttonText}>Click the cool button</Text>
</TouchableOpacity>
</View>
);
}
const styles = StyleSheet.create({
container: {
justifyContent: 'flex-start',
backgroundColor: '#F2F2F7FF',
paddingLeft: 30,
},
title: {
fontSize: 28,
},
buttonText: {
fontWeight: 'bold',
},
});